6.2 KiB
6.2 KiB
id, challengeType, videoUrl, localeTitle
id | challengeType | videoUrl | localeTitle |
---|---|---|---|
587d8256367417b2b2512c7a | 1 | 在二叉搜索树中查找最小值和最大值 |
Description
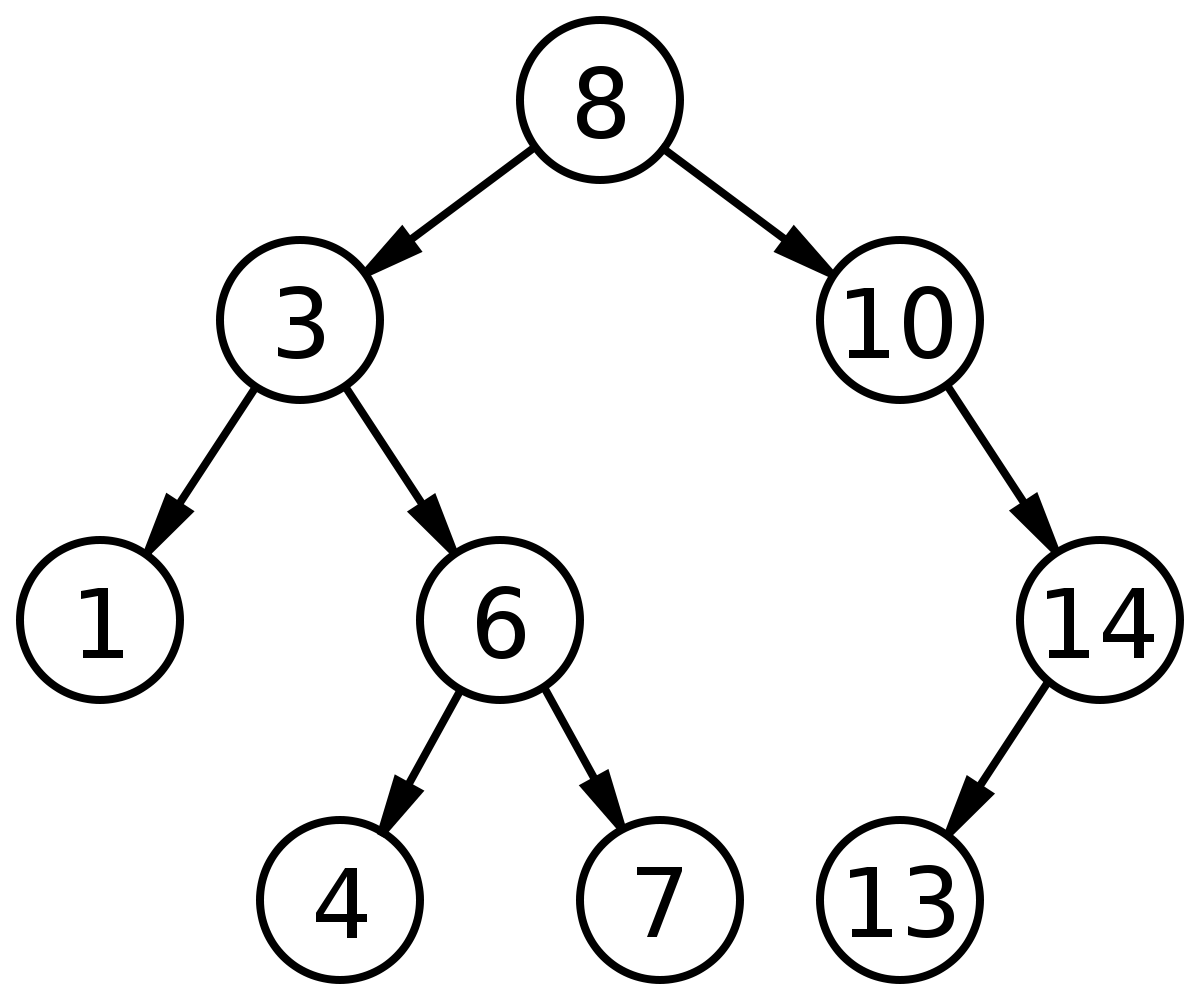
findMin
和findMax
。这些方法应返回二叉搜索树中保存的最小值和最大值(不用担心现在向树中添加值,我们在后台添加了一些值)。如果遇到困难,请反思二进制搜索树必须为true的不变量:每个左子树小于或等于其父树,每个右子树大于或等于其父树。我们还要说我们的树只能存储整数值。如果树为空,则任一方法都应返回null
。 Instructions
Tests
tests:
- text: 存在<code>BinarySearchTree</code>数据结构。
testString: assert((function() { var test = false; if (typeof BinarySearchTree !== 'undefined') { test = new BinarySearchTree() }; return (typeof test == 'object')})());
- text: 二叉搜索树有一个名为<code>findMin</code>的方法。
testString: assert((function() { var test = false; if (typeof BinarySearchTree !== 'undefined') { test = new BinarySearchTree() } else { return false; }; return (typeof test.findMin == 'function')})());
- text: 二叉搜索树有一个名为<code>findMax</code>的方法。
testString: assert((function() { var test = false; if (typeof BinarySearchTree !== 'undefined') { test = new BinarySearchTree() } else { return false; }; return (typeof test.findMax == 'function')})());
- text: <code>findMin</code>方法返回二叉搜索树中的最小值。
testString: assert((function() { var test = false; if (typeof BinarySearchTree !== 'undefined') { test = new BinarySearchTree() } else { return false; }; if (typeof test.findMin !== 'function') { return false; }; test.add(4); test.add(1); test.add(7); test.add(87); test.add(34); test.add(45); test.add(73); test.add(8); return test.findMin() == 1; })());
- text: <code>findMax</code>方法返回二叉搜索树中的最大值。
testString: assert((function() { var test = false; if (typeof BinarySearchTree !== 'undefined') { test = new BinarySearchTree() } else { return false; }; if (typeof test.findMax !== 'function') { return false; }; test.add(4); test.add(1); test.add(7); test.add(87); test.add(34); test.add(45); test.add(73); test.add(8); return test.findMax() == 87; })());
- text: <code>findMin</code>和<code>findMax</code>方法为空树返回<code>null</code> 。
testString: assert((function() { var test = false; if (typeof BinarySearchTree !== 'undefined') { test = new BinarySearchTree() } else { return false; }; if (typeof test.findMin !== 'function') { return false; }; if (typeof test.findMax !== 'function') { return false; }; return (test.findMin() == null && test.findMax() == null) })());
Challenge Seed
var displayTree = (tree) => console.log(JSON.stringify(tree, null, 2));
function Node(value) {
this.value = value;
this.left = null;
this.right = null;
}
function BinarySearchTree() {
this.root = null;
// change code below this line
// change code above this line
}
After Test
console.info('after the test');
Solution
// solution required
/section>